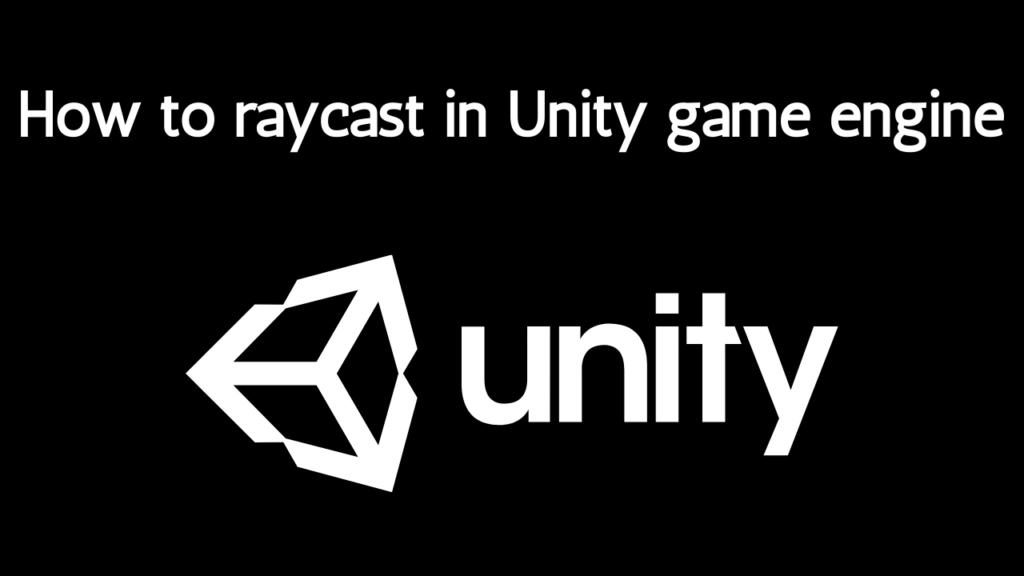
Introduction
Raycasting is a fundamental technique in game development, especially when working with 3D environments. Whether you’re building a complex shooter, a detailed puzzle game, or an immersive VR experience, understanding how to raycast effectively in Unity can take your projects to the next level. In this article, we’ll dive deep into the world of raycasting, exploring its concepts, applications, and best practices to help you become proficient in using this powerful tool.
Getting Started with Raycasting in Unity
Setting Up Your Unity Project
Before you can start raycasting, you need to have a Unity project ready to go. If you’re new to Unity, don’t worry! Setting up a project is straightforward. Simply open Unity, create a new project, and ensure you have a basic scene with some 3D objects to interact with. This could be as simple as a few cubes and a plane to serve as your ground.
Understanding the Unity Editor
Familiarize yourself with the Unity Editor, as it will be your primary workspace. The Scene view, Game view, Hierarchy, Inspector, and Project windows are essential for navigating and managing your game objects and scripts. Understanding these elements will make implementing raycasting much easier.
Basic Raycasting Concepts

What is a Ray?
A ray is essentially a straight line that starts at a specific point and extends infinitely in a particular direction. In Unity, rays are used to detect objects in the 3D space by “shooting” them from a starting point and seeing what they hit.
Understanding RaycastHit
When a ray intersects with an object, Unity provides information about the collision through a RaycastHit
object. This includes details such as the point of impact, the normal at the impact point, and the collider that was hit. This information is crucial for determining how to respond to the raycast.
Implementing Raycasting in Unity
Writing Your First Raycast Script
Let’s get hands-on with a basic raycasting script. Create a new C# script in Unity and name it RaycastExample
. Here’s a simple script to get you started:
Csharp Code
using UnityEngine;
public class RaycastExample : MonoBehaviour
{
void Update()
{
if (Input.GetMouseButtonDown(0))
{
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
RaycastHit hit;
if (Physics.Raycast(ray, out hit))
{
Debug.Log("Hit " + hit.collider.name);
}
}
}
}
This script casts a ray from the camera to where the mouse is clicked and logs the name of the object it hits.
Testing Your Raycast
Attach the RaycastExample
script to a GameObject in your scene, such as the Main Camera. Press play and click on objects in your scene to see the raycast in action. The console should display the names of the objects you’re clicking on.
Advanced Raycasting Techniques

Multi-Raycasting
Sometimes, one ray isn’t enough. You might need to cast multiple rays in different directions or use them in sequence to achieve more complex interactions. Unity provides methods like Physics.RaycastAll
to handle multiple hits in a single cast.
Layer Masks and Filtering
You can use layer masks to filter which objects a ray can hit. This is useful for ignoring certain objects or layers when casting rays. Here’s an example:
Csharp Code
int layerMask = 1 << 8;
if (Physics.Raycast(ray, out hit, Mathf.Infinity, layerMask))
{
Debug.Log("Hit " + hit.collider.name);
}
Raycasting with Physics
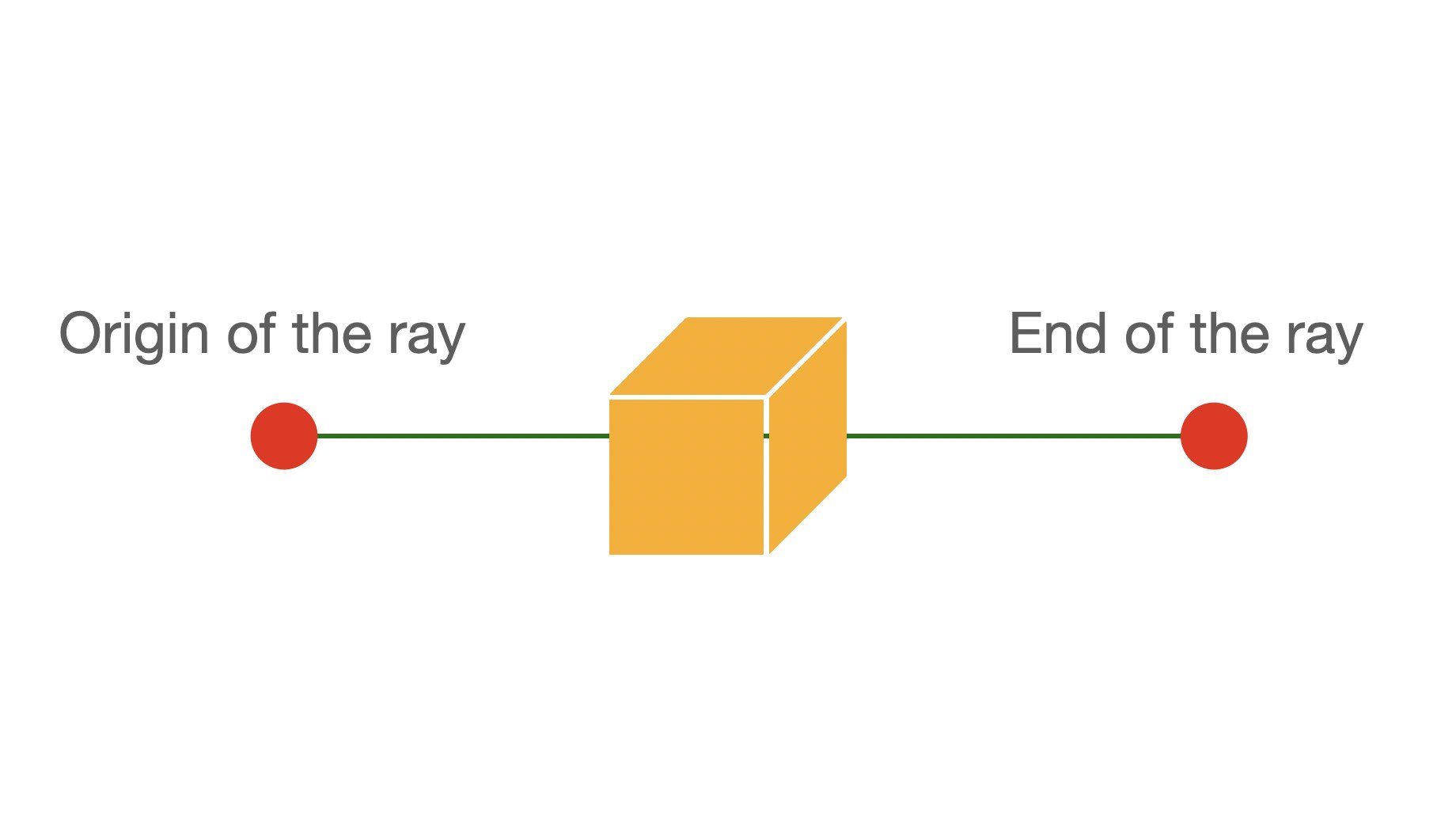
Using Raycasting for Collision Detection
Raycasting is often used for collision detection in games. By casting rays in various directions, you can determine if an object is about to collide with another object and react accordingly.
Raycasting and Rigidbody Interactions
Combining raycasting with Rigidbody physics can create realistic interactions. For example, you can use raycasts to determine where a projectile should land and apply forces to Rigidbodies based on raycast hits.
Raycasting for Interaction

Click Detection
Raycasting is perfect for detecting clicks on objects. You can use the RaycastHit
information to trigger events or actions based on what the player clicks on.
Object Selection and Highlighting
Use raycasting to allow players to select and highlight objects in your game. This can be done by changing the material or adding visual effects to the object that the raycast hits.
Raycasting for AI and Navigation
Line of Sight Detection
AI characters often use raycasting to determine if they can see the player or other objects. This helps in creating realistic behaviors, like hiding or seeking cover.
Navigation and Pathfinding
Raycasting assists in navigation and pathfinding by checking for obstacles along a path. This ensures that AI characters can move around the environment without getting stuck.
Optimizing Raycasting Performance
Reducing Raycasting Overhead
Raycasting can be performance-intensive, especially in large scenes. Optimize by reducing the frequency of raycasts or limiting the distance they travel.
Efficient Use of Raycasting in Large Scenes
Use spatial partitioning techniques like Octrees or Grid-based systems to minimize the number of objects that need to be checked by each raycast.
Debugging Raycasting Issues
Common Raycasting Problems
Common issues with raycasting include rays not hitting expected objects or hitting unintended ones. These problems often stem from incorrect layer masks, colliders, or ray directions.
Debugging Techniques and Tools
Unity’s debugging tools, such as Debug.DrawRay
, can help visualize raycasts in the Scene view. This makes it easier to see what your rays are hitting and adjust your scripts accordingly.
Practical Examples of Raycasting
Raycasting in First-Person Shooters
In first-person shooters, raycasting is used to detect what the player is aiming at, determine hit points for bullets, and apply damage to targets.
Raycasting in Puzzle Games
Puzzle games often use raycasting to detect player interactions with objects, such as clicking to move pieces or triggering events when objects align.
Raycasting in VR and AR
![a) Ray-casting. (b) Bubble Cursor [12]. (c) Ray-casting with bubble... | Download Scientific Diagram](https://www.researchgate.net/publication/342061083/figure/fig1/AS:996815272951808@1614670689405/a-Ray-casting-b-Bubble-Cursor-12-c-Ray-casting-with-bubble-mechanism-The-ray.png)
Implementing Raycasting for VR Interactions
In VR, raycasting helps with interaction by allowing players to point and select objects using their VR controllers. This creates an intuitive way to interact with the virtual environment.
Raycasting in Augmented Reality
For AR applications, raycasting is used to detect surfaces and place virtual objects in the real world, enhancing the interactive experience.
Raycasting in Multiplayer Games

Synchronizing Raycasts Across Clients
In multiplayer games, it’s crucial to synchronize raycast results across all clients to ensure consistency. This can involve networked raycasts and authoritative server checks.
Handling Latency and Prediction
Managing latency is key in multiplayer raycasting. Techniques like client-side prediction and server reconciliation help maintain a smooth experience for all players.
Extending Raycasting with Custom Scripts
Creating Custom Raycasting Functions
You can extend Unity’s raycasting capabilities by writing custom functions that add new features or handle specific cases not covered by default methods.
Integrating Raycasting with Other Systems
Integrate raycasting with other game systems, such as animations, sound effects, and user interfaces, to create more immersive and interactive experiences.
Conclusion
Raycasting is an incredibly versatile tool in Unity, essential for everything from basic interactions to complex AI behaviors. By understanding and mastering raycasting, you can enhance your game’s interactivity, realism, and overall player experience. So, start experimenting with raycasting in your projects, and watch as your game development skills reach new heights!
FAQs
1. What is raycasting in Unity? Raycasting in Unity is a technique used to detect objects in a 3D environment by projecting an invisible ray from a point and checking what it intersects with.
2. How do I use raycasting for click detection? You can use raycasting for click detection by casting a ray from the camera to the mouse position and checking if it hits any objects.
3. Can raycasting be used for AI in Unity? Yes, raycasting is commonly used for AI to determine line of sight, detect obstacles, and assist in navigation and pathfinding.
4. How do I optimize raycasting in a large scene? Optimize raycasting by reducing its frequency, limiting the distance of rays, and using spatial partitioning techniques to minimize the number of objects checked.
5. What are some common issues with raycasting? Common issues include rays not hitting expected objects, incorrect layer masks, and problems with ray directions. Debugging tools like Debug.DrawRay
can help visualize and solve these problems.