Do You Need to Learn C# for Unity?
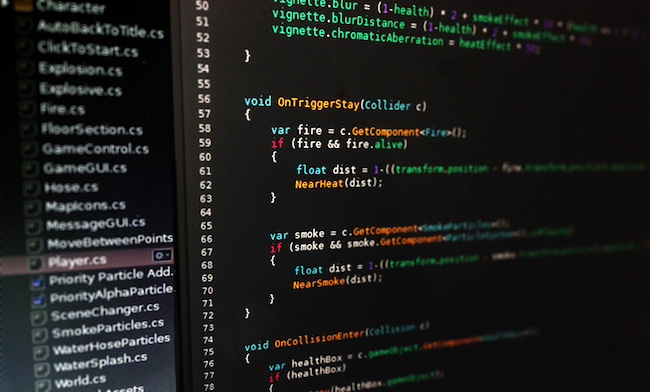
Introduction
Unity is one of the most popular game development engines in the world, widely used for creating both 2D and 3D games. It provides a comprehensive set of tools for developers, including a powerful scripting API that allows for extensive customization and control over game behavior. The primary language used for scripting in Unity is C#, which raises the question: do you need to learn C# for Unity? This article will delve into the necessity of learning C# for Unity, its benefits, and how it integrates into the game development process. Do You Need to Learn C# for Unity? is the main question.
Overview of Unity
Unity is a versatile game engine developed by Unity Technologies, first released in 2005. It supports a wide range of platforms including PC, consoles, mobile devices, and even AR/VR environments. Unity is known for its user-friendly interface, extensive asset store, and strong community support, making it a preferred choice for both indie developers and large studios.
The Role of C# in Unity
C# is the primary programming language used in Unity for scripting. It allows developers to control the behavior of game objects, implement game logic, and create complex interactions within the game. C# is chosen for its simplicity, powerful features, and performance. Unity’s scripting API is designed to work seamlessly with C#, providing a robust framework for game development.
Alternative Scripting Languages in Unity
While C# is the dominant language for Unity scripting, other languages like JavaScript (UnityScript) and Boo were previously supported. However, Unity has since deprecated these languages, focusing exclusively on C# for scripting. This shift underscores the importance of C# in modern Unity development.
Learning C# for Unity

Basics of C#
To start with Unity, a fundamental understanding of C# is essential. Here are the key concepts to grasp:
- Variables and Data Types: Understanding different data types (int, float, string, etc.) and how to use them.
- Control Structures: Mastering if statements, loops (for, while), and switch cases.
- Functions and Methods: Learning to write reusable code blocks.
- Object-Oriented Programming (OOP): Understanding classes, objects, inheritance, and polymorphism.
- Error Handling: Using try-catch blocks for debugging and error management.
Advanced C# Concepts
For more advanced Unity projects, deeper knowledge of C# is required:
- Delegates and Events: Essential for handling events and callbacks in Unity.
- Generics: Writing flexible and reusable code.
- LINQ (Language Integrated Query): Querying collections in a concise and readable manner.
- Asynchronous Programming: Using async and await for managing asynchronous operations.
- Design Patterns: Applying common design patterns like Singleton, Observer, and Factory to solve common problems.
C# in Unity: A Practical Approach
Setting Up Your Development Environment
To start scripting in Unity, you need to set up a development environment. This typically involves:
- Installing Unity: Download and install the Unity Hub, then install the Unity Editor.
- Setting Up an IDE: Visual Studio is the recommended Integrated Development Environment (IDE) for C#. Unity usually installs Visual Studio alongside the Editor, but it can also be downloaded separately.
- Creating a New Project: Open Unity, create a new project, and familiarize yourself with the interface.
Writing Your First Script
Creating and attaching your first C# script in Unity is straightforward:
- Create a New Script: In the Unity Editor, navigate to the Project window, right-click, and select Create > C# Script. Name your script (e.g., “PlayerController”).
- Open the Script: Double-click the script to open it in Visual Studio.
- Write Code: Start by writing a simple script to move a game object. For example
csharp
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float speed = 5.0f;
void Update()
{
float moveHorizontal = Input.GetAxis("Horizontal");
float moveVertical = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveHorizontal, 0.0f, moveVertical);
transform.Translate(movement * speed * Time.deltaTime);
}
}
- Attach the Script: Attach the script to a game object in the Unity Editor by dragging it from the Project window onto the desired object in the Hierarchy window.
Common C# Scripts in Unity
Some common scripts you might write in Unity include:
- Player Movement: Controlling the movement of a player character.
- Enemy AI: Implementing basic AI behaviors for enemies.
- Camera Control: Creating dynamic camera systems.
- UI Management: Handling user interface interactions and updates.
- Physics Interactions: Managing collisions and physics-based actions.
Benefits of Learning C# for Unity
Learning C# for Unity offers numerous benefits:
- Job Opportunities: Many game development studios require knowledge of C# and Unity.
- Versatility: C# is used in various applications beyond game development, such as software development and enterprise solutions.
- Community Support: A large community of developers and extensive resources are available for learning and troubleshooting.
- Performance: C# offers excellent performance, crucial for developing smooth and efficient games.
- Integration with .NET: C# is part of the .NET ecosystem, allowing access to a wide range of libraries and tools.
Challenges of Learning C# for Unity
Despite its benefits, learning C# for Unity can present challenges:
- Steep Learning Curve: For beginners, C# and Unity can be overwhelming due to their complexity.
- Debugging: Debugging scripts in Unity requires a good understanding of both C# and the Unity engine.
- Continuous Learning: Both C# and Unity are continuously evolving, requiring ongoing learning and adaptation.
- Performance Optimization: Writing efficient C# code for high-performance games requires experience and deep knowledge of the language and engine.
Resources for Learning C#
Online Courses
- Coursera: Offers comprehensive courses on C# and Unity.
- Udemy: Numerous courses ranging from beginner to advanced levels.
- Pluralsight: Detailed tutorials and courses for professional development.
Books
- “C# in Depth” by Jon Skeet: A deep dive into C#.
- “Learning C# by Developing Games with Unity” by Harrison Ferrone: A practical guide focused on game development.
- “Pro Unity Game Development with C#” by Alan Thorn: Advanced techniques for professional game developers.
Tutorials
- Unity Learn: Official tutorials from Unity Technologies.
- Brackeys: Popular YouTube channel with beginner-friendly tutorials.
- CodeMonkey: Tutorials focusing on C# scripting in Unity.
Tips for Mastering C#
Practice Regularly
Regular practice is essential for mastering C#. Set aside dedicated time each day to code and experiment with different concepts.
Build Small Projects
Start with small projects to apply what you’ve learned. Gradually increase the complexity as you become more comfortable with C# and Unity.
Join a Community
Join online communities and forums such as the Unity forums, Reddit, or Discord servers. Engaging with other developers can provide support, feedback, and new learning opportunities.
FAQs about C# and Unity
Q: Do I need to be an expert in C# to use Unity?
A: No, you don’t need to be an expert. A basic understanding of C# is sufficient to get started with Unity. As you work on more complex projects, your C# skills will naturally improve.
Q: Can I use other languages in Unity?
A: Unity primarily supports C# for scripting. While other languages like UnityScript and Boo were previously supported, they are now deprecated.
Q: How long does it take to learn C# for Unity?
A: The time it takes to learn C# varies depending on your prior programming experience. Beginners might take a few months to become comfortable, while those with experience in other languages might pick it up more quickly.
Q: Are there any shortcuts to learning C# for Unity?
A: There are no shortcuts, but using structured resources like online courses, tutorials, and books can streamline the learning process.
Q: What are the best practices for writing C# scripts in Unity?
A: Follow best practices such as keeping code modular, using comments and documentation, adhering to naming conventions, and optimizing performance.
Conclusion
Learning C# is crucial for unlocking the full potential of Unity. While it might seem daunting at first, the benefits far outweigh the challenges. By leveraging the resources and tips provided in this article, you can master C# and create amazing games with Unity. Dive in, start coding, and join the thriving community of Unity developers.